CodeIgniter 4 is a lightweight and powerful PHP framework that developers love for building dynamic web applications. However, there are times when PHP alone might not be enough. Python, with its rich ecosystem of libraries and tools, is often better suited for tasks like machine learning, data analysis, or working with advanced libraries like LangChain or vector databases such as Chroma. By integrating Python into your CodeIgniter 4 project, you can combine the strengths of both languages to create more versatile and powerful applications.
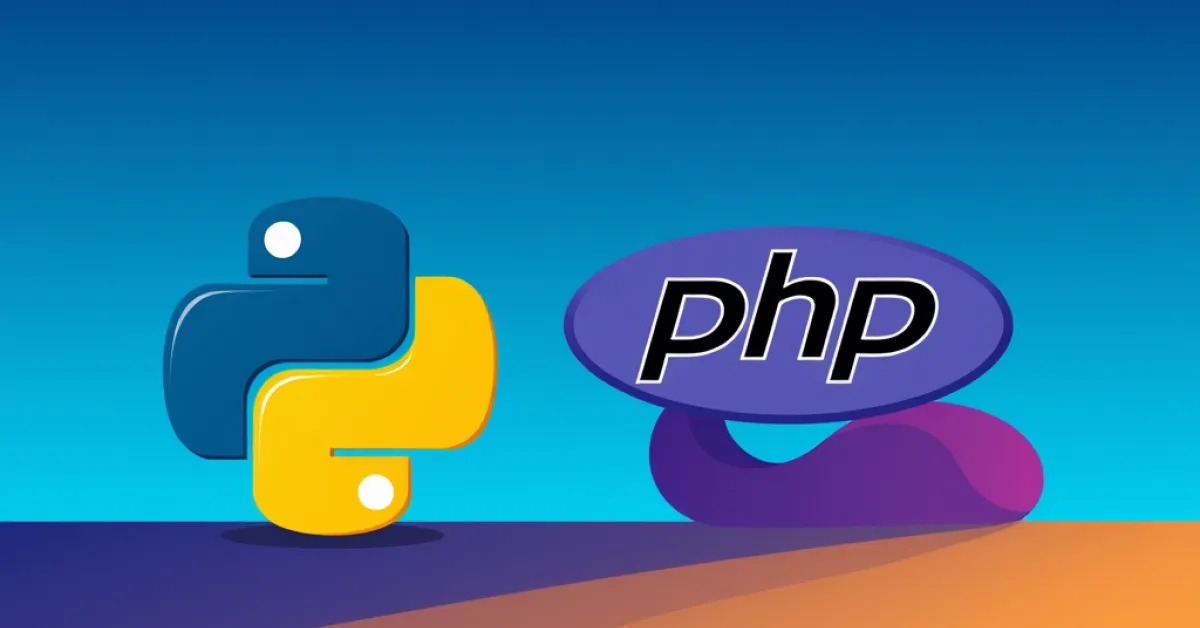
This guide will walk you through how to execute Python scripts from CodeIgniter 4, receive their output, and use it effectively. We’ll also explore common use cases and best practices for this integration.
Why Integrate Python with CodeIgniter 4?
While PHP is excellent for web development, Python shines in areas like:
- Machine Learning and AI: Python libraries like TensorFlow, PyTorch, and LangChain make it ideal for AI tasks.
- Data Analysis: Tools like Pandas and NumPy simplify data manipulation.
- Vector Databases: Python works seamlessly with vector databases like Chroma for advanced search and retrieval tasks.
- Automation: Python excels at automating repetitive tasks.
By integrating Python into your CodeIgniter 4 project, you can offload complex tasks to Python while using PHP for web-related functionality. For example, you might use Python to process user-uploaded data, perform AI-based predictions, or query a vector database, and then display the results in your PHP-based web app.
Methods to Integrate Python with CodeIgniter 4
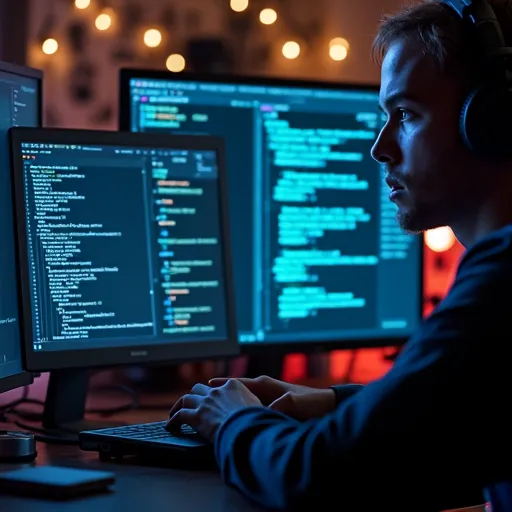
Visualising the synergy between Python’s prowess in AI and data analysis and CodeIgniter 4’s PHP foundation.
There are several ways to execute Python scripts and retrieve their output in CodeIgniter 4. Each method has its own use cases, advantages, and considerations.
1. Using PHP’s shell_exec
Function
The simplest way to run a Python script from CodeIgniter is by using PHP’s shell_exec
function. This function allows you to execute shell commands, including Python scripts, and capture their output.
Example
// Controller method in CodeIgniter
public function runPythonScript()
{
$scriptPath = WRITEPATH . 'scripts/my_script.py'; // Path to your Python script
$output = shell_exec('python3 ' . escapeshellarg($scriptPath));
echo $output; // Display the output from the Python script
}
Key Points:
- Script Location: Place your Python scripts in the
/writable/scripts
directory of your CodeIgniter project. This keeps them organised and ensures they are writable by the server. - Security: Always sanitise inputs to prevent command injection. Use
escapeshellarg()
to safely pass arguments to the script. - Dependencies: Ensure Python and any required libraries are installed on your server.
Common Use Case:
- Running a Python script that processes data and returns results, such as querying a vector database like Chroma or generating AI-based responses using LangChain.
2. Using REST APIs
For more complex or scalable integrations, you can set up a REST API in Python and communicate with it from CodeIgniter. This approach decouples your Python logic from your PHP application, making it easier to maintain and scale.
Steps:
- Create a Python REST API:
- Use a framework like Flask or FastAPI to create an API that performs specific tasks.
from flask import Flask, request, jsonify
app = Flask(__name__)
@app.route('/process', methods=['POST'])
def process_data():
data = request.json
result = {"message": f"Hello, {data['name']}!"}
return jsonify(result)
if __name__ == '__main__':
app.run(port=5000)
- Consume the API in CodeIgniter:
- Use CodeIgniter’s HTTP client to send requests to the Python API and handle the responses.
public function callPythonApi()
{
$client = \Config\Services::curlrequest();
$response = $client->post('http://localhost:5000/process', [
'json' => ['name' => 'CodeIgniter']
]);
$data = json_decode($response->getBody(), true);
echo $data['message']; // Output: Hello, CodeIgniter!
}
Key Points:
- Flexibility: This method allows you to use Python for complex tasks while keeping your CodeIgniter app focused on web development.
- Scalability: You can deploy the Python API on a separate server or container for better performance.
Common Use Case:
- Using Python for tasks like natural language processing with LangChain or querying a vector database, and returning the results to CodeIgniter.
3. Using Message Queues
For asynchronous or background tasks, message queues like RabbitMQ or Redis are a great option. This method is ideal for handling long-running Python tasks without blocking your PHP application.
Steps:
- Set Up a Message Queue:
- Use a library like Celery in Python to process tasks from the queue.
from celery import Celery
app = Celery('tasks', broker='redis://localhost:6379/0')
@app.task
def add(x, y):
return x + y
- Send Messages from CodeIgniter:
- Use a PHP library like
php-amqplib
to send messages to the queue.
- Use a PHP library like
public function sendToQueue()
{
$connection = new AMQPStreamConnection('localhost', 5672, 'guest', 'guest');
$channel = $connection->channel();
$channel->queue_declare('task_queue', false, true, false, false);
$data = json_encode(['x' => 5, 'y' => 10]);
$msg = new AMQPMessage($data, ['delivery_mode' => 2]);
$channel->basic_publish($msg, '', 'task_queue');
echo "Task sent to queue!";
}
- Retrieve Results:
- Once the Python worker processes the task, it can store the results in a database or send them back to CodeIgniter via a callback.
Key Points:
- Asynchronous Processing: This method is ideal for tasks that take time, such as training a machine learning model or processing large datasets.
- Scalability: Message queues can handle high volumes of tasks efficiently.
Common Use Case:
- Offloading heavy computations, like vector similarity searches in Chroma, to Python while keeping your PHP app responsive.
Best Practices for Integration
Organise Your Scripts:
- Store Python scripts in the
/writable/scripts
directory of your CodeIgniter project for easy access and management.
- Store Python scripts in the
Environment Configuration:
- Ensure both PHP and Python environments are properly configured on your server.
- Use virtual environments in Python to manage dependencies.
Security:
- Validate and sanitise all inputs to prevent security vulnerabilities.
- Restrict access to sensitive scripts and APIs.
Error Handling:
- Implement robust error handling in both your PHP and Python code to ensure smooth operation.
Performance Optimisation:
- Minimise delays by optimising your Python scripts and using caching where possible.
Conclusion
Integrating Python into a CodeIgniter 4 project opens up a world of possibilities. Whether you’re leveraging Python’s powerful libraries for machine learning, querying vector databases like Chroma, or automating tasks, the combination of PHP and Python can create highly functional and efficient applications.
By using methods like shell_exec
, REST APIs, or message queues, you can tailor the integration to your specific needs. With careful planning, attention to security, and proper organisation, you can harness the best of both worlds to build applications that are both dynamic and intelligent.